Master Modern JavaScript Fast: A Comprehensive Guide

javascript console.log("Hello, world!");
You can run this code snippet in your web browser's console. To do this, open your web browser and press Ctrl+Shift+J (Windows) or Cmd+Option+J (Mac). Then, paste the code snippet into the console and press Enter.
The following are some of the basic concepts that you need to understand in order to use JavaScript effectively:
4.4 out of 5
Language | : | English |
File size | : | 13336 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 307 pages |
- Variables are used to store data in JavaScript. You can declare a variable using the
let
keyword. For example, the following code snippet declares a variable calledname
and assigns it the value "John Doe":
javascript let name = "John Doe";
- Data types define the type of data that a variable can store. JavaScript has several built-in data types, including:
- Number: Stores numeric values.
- String: Stores text values.
- Boolean: Stores true or false values.
- Object: Stores collections of key-value pairs.
- Array: Stores ordered lists of values.
- Operators are used to perform operations on variables. JavaScript has a variety of operators, including:
- Arithmetic operators: Used to perform arithmetic operations, such as addition, subtraction, and multiplication.
- Comparison operators: Used to compare values.
- Logical operators: Used to combine multiple conditions.
- Control flow statements allow you to control the flow of execution in your JavaScript code. The most common control flow statements are:
- If statements: Used to execute code only if a certain condition is met.
- Switch statements: Used to execute different code depending on the value of a variable.
- For loops: Used to iterate over a sequence of values.
- While loops: Used to execute code while a condition is met.
Once you have a good understanding of the basics, you can start learning more intermediate concepts. These concepts include:
- Functions are used to group code together and perform specific tasks. You can declare a function using the
function
keyword. For example, the following code snippet declares a function calledgreet
that takes a name as an argument and prints a greeting to the console:
javascript function greet(name){console.log("Hello, " + name + "!"); }
- Objects are used to store data in a structured way. You can create an object using the
{}
notation. For example, the following code snippet creates an object calledperson
that stores the name, age, and occupation of a person:
javascript const person = { name: "John Doe", age: 30, occupation: "Software engineer" };
- Arrays are used to store ordered lists of values. You can create an array using the
[]
notation. For example, the following code snippet creates an array callednumbers
that stores the numbers 1, 2, 3, 4, and 5:
javascript const numbers = [1, 2, 3, 4, 5];
* **Event listeners** are used to listen for events on web pages. For example, you can use an event listener to listen for click events on a button. When the button is clicked, the event listener will trigger a function that performs a specific task. <h2>Advanced JavaScript Concepts</h2> Once you have a good understanding of intermediate concepts, you can start learning more advanced concepts. These concepts include: * **Asynchronous programming** is used to handle operations that take a long time to complete, such as fetching data from a server. Asynchronous programming allows you to continue executing code while the long-running operation is in progress. * **Promises** are used to handle asynchronous operations. A promise represents the eventual result of an asynchronous operation. You can use promises to chain multiple asynchronous operations together. * **Callbacks** are functions that are passed as arguments to other functions. Callbacks are often used to handle the results of asynchronous operations. * **Modules** are used to organize JavaScript code into reusable units. You can create a module by exporting a set of functions, classes, or variables. You can then import modules into other JavaScript files to use their functionality. <h2>Real-World JavaScript Examples</h2> The following are some real-world examples of how JavaScript is used: * **Interactive web applications:** JavaScript is used to create interactive web applications, such as online games, chat applications, and social media platforms. * **Dynamic websites:** JavaScript is used to create dynamic websites that can change their content and appearance based on user input. * **Mobile apps:** JavaScript is used to create mobile apps using frameworks such as React Native and Ionic. * **Server-side development:** JavaScript can be used to develop server-side applications using frameworks such as Node.js and Express. JavaScript is a powerful and versatile programming language that is essential for modern web development. By mastering modern JavaScript, you will be able to create your own web applications and websites, and you will be able to contribute to the development of the web.</body></html>
4.4 out of 5
Language | : | English |
File size | : | 13336 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 307 pages |
Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
Fiction
Non Fiction
Romance
Mystery
Thriller
SciFi
Fantasy
Horror
Biography
Selfhelp
Business
History
Classics
Poetry
Childrens
Young Adult
Educational
Cooking
Travel
Lifestyle
Spirituality
Health
Fitness
Technology
Science
Arts
Crafts
DIY
Gardening
Petcare
Marvin Valerie Georgia
Barbara Decker
Og Mandino
Norton Juster
Emma Crewe
Barry Dainton
Chris Worfolk
Adam Enaz
Carl Jones
Sally Clarkson
Shuai Huang
Robert Fritz
Stephen Brennan
Sheila Lamb
Edward J Larson
Lucy Letcher
Jennifer Greene
Timothy Phelps
Sarah Moore
Amber Fox
Seth Kugel
Adam Braus
Sean Lewis
Agustin Fuentes
David Simon
Douglas W Hubbard
Christian Beamish
Martha C Nussbaum
Abigail Owen
Kate Rope
Caroline Kaufman
Lillian Cumic
Jennifer Shannon
G Bailey
Charles C Patrick
Tony Soper
Virginia Smith Harvey
Steve Mcmenamin
R L Stine
Chuck Callaway
Matt Owens
Axie Oh
Jacob Neumann
Harley Rustad
Abby Sunderland
Monica Sorrenson
Terry Palechuk
Abigail Marshall
Sharon Boyd
Marlene Wagman Geller
Stephanie Zeiss
Abigail Pogrebin
Tim Jarvis
Bill Mckibben
Jay Ruud
Phil Gaimon
Rosie Pope
Joe Simpson
Walter Browder
Rob Gray
Kemi Iwalesin
Alicia Silverstone
Stefan Ball
Allan Sand
Mikki Daughtry
John Taylor
Sarah K L Wilson
Jack Moore
Paula Deen
Irene Lewis Mccormick
T L Lowery
Peter Sagal
Jack Cavanaugh
Michelle Hodkin
Mary Wong
Jeremy Paxman
Steve Rosenberg
Reinette Biggs
Ryan Smithson
Adam Boduch
Michel Roy
Carley Roney
Ilsa J Bick
Jennifer Ackerman
Helen Scales
Irakli Makharadze
Gail Buckland
Gerry Donohue
Abigail Melton
Hans C Ohanian
Vivian Gussin Paley
Meg Keys
Daphne Poltz
William Hamilton Gibson
Jon Butterworth
Stephen Gray
Abigail Hing Wen
James Heberd
Erik Scott De Bie
Brad Brewer
Michael Johnson
Rania Abouzeid
Ronald Kaine
Zoyla Arana
Carolyn Jessop
Charles H Kraft
Megan Lane
Brian L Gorman
David Lipsky
Winston Chang
Adam Benshea
Sheri Van Dijk
Brian Hoggard
Adam Frank
Kristin Berry
Steven Alan Childress
Lisa M Given
Stephanie Puglisi
Christopher Black
Aliza Green
Christina Hoff Sommers
Peter Harrison
Debbie Elicksen
Adam Lazarus
Kevin A Morrison
Lisa Robertson
Katie Lear
Farah Shabazz Ii
Barbara Fox
Adam H Balen
Paul Schneider
Barry Robinson
Richard Lighthouse
Maya Lang
Moon Ho Jung
Dmv Test Bank
Abby Hafer
Charlie Barker
Erika Fatland
Gavin D J Harper
Christopher S Stewart
Aaron J Perry
James Surowiecki
Aiden Thomas
Sheldon Axler
Tom Migdalski
Arthur Turrell
Abbas Kazerooni
Bill Loguidice
Barbara Natterson Horowitz
Lois G Schwoerer
Adam Lashinsky
Chaz Scoggins
Jay Dawes
Helen C Rountree
John Caig
Michael Gruenbaum
Elena Aguilar
Eduardo Duran
Phong Thong Dang
Abby Haight
Craig Timberg
Betsy Miller
Ann Mariah Cook
Tamora Pierce
Wayne Westcott
Carmen Moreno
Papus
Mark Januszewski
Sherri Granato
Brian Greene
Jeremy Shinewald
Jason Korol
William Deresiewicz
Roman Dial
Asato Asato
Neil Oliver
Andy Dumas
Kathy Koch Phd
Michael Crawley
Ned Johnson
Abraham Silberschatz
Ralph Villiger
Sam J Miller
Pamela Druckerman
Jeremy Miles
Gregor Clark
Alan Robertson
Abby Mcallister
Suzanne Corkin
Al Barkow
Achim K Krull
Gladys Chepkirui Ngetich
Pearson Education
Sissy Goff
Lisa Heffernan
Robert Reid
Bobby Reyes
Maureen Duffin Ward
Renda Dionne Madrigal
Beth Newell
Genius Reads
Natasha Preston
Rick Sekuloski
Aaron Lee Johnson
Aaron Hahn
Abigail Tucker
Abigail Alling
Cassandra Eason
Tom Mccarthy
Rex Ogle
Adam J Cox
Marc Dando
Baba Ifa Karade
Scott Butler
Rachael Ray
Christophe Jaffrelot
Eugene H Merrill
Randall Hyde
Laura Gao
Adam Chandler
Ian Davis
Shari Eskenas
Anthony Wilkenson
Chashiree M
Hillary Allen
Martha Gellhorn
Ken Springer
Lenyfer Garrido
Vivienne Sanders
Horace Kephart
Jeff Bauman
Lynn E Ponton
Richard Sattora
Nancy Mohrbacher
Daniel Carter Beard
Paul Mclerran
Louise Pickford
Zoe Hamlet Silva
Stacy Eaton
Deborah Beck Busis
Al Desetta
Tom Jackson
Liza Angela
Kel Carpenter
Melodie M Davis
Claire Ahn
David Winner
Miranda Kenneally
Ariana Eagleton
Achille Rubini
Abbey Curran
Aaron Oster
Alifya And Umesh Mohite
George John Romanes
Winifred Conkling
Susan Walton
Cornel West
Larry Carpenter
Adam Kimelman
Michael L Bloomquist
Claire Santry
Iain Highfield
Jim Willis
Marie Cirano
Lin Wellford
Vince Kotchian
Charles L Byrne
Dan Shideler
Al Yellon
Shannon Hale
Chris Jericho
Lindsey Ellison
Michael Baigent
David Feddes
Jasna Tuta
P S Page
Lucio Russo
Eze Ugbor
Maxine Levaren
Justin Driver
John Kean
Andrea M Nelson Royes
Steve Griffith
Bathroom Readers Institute
Peter David
Gil Capps
Emily K Neuburger
Tim Larkin
O Thomas Gift
Casey Robson
Israel Finkelstein
Programming Languages Academy
Steve Roper
Man Kam Lo
Jennifer L Hunt
Kim Gosselin
Laura Ray
Sarah Luddington
Laurence Steinberg
Jimmy Houston
J Robert King
Miriam Forman Brunell
David Rensin
Adam Galinsky
Judi Kesselman Turkel
Sophie Mccartney
George J Hademenos
Adam Becker
Scott Parsons
Abdul Foster
M J Parisian
Susan Zeppieri
Amby Burfoot
Stuart Firestein
Adam D Scott
Katrina Kahler
Emily J Taylor
Lisa Latimer
Xiuhtezcatl Martinez
Adam Koch
Kirk Bailey
Robert Allans
Garrett Mcnamara
Adam Freeman
Lisa M Schab
Kit Bauman
John Hancock
Amelia Whitmore
Jenny Han
Lavinia Collins
Sasha Abramsky
Abridged Ed Edition Kindle Edition
Matt Warshaw
Rory Miller
Conrad Bauer
Gerald A Voorhees
An American Citizen
Mark Strom
Gary Sakuma
Lisa Pineda
Richard Martin
Annie Nicholas
Ron Jones
Nick Holt
Dan Morris
Emily Suzanne Clark
Sarah Maslin Nir
Fred Fields
Chella Quint
David Warriner
Ed Engle
Felice Fox
Clara Shaper
Taylan Hoca
Aaron Graves
Devika Primic
Gayle Forman
Al Baird
Fumio Sasaki
Glen Finland
Charles Thomas Jr
Barbara A Lewis
Jane Gross
Ken Retallic
Darrin Bergman
Gloria Atanmo
Dick Edie
Todd Graves
Benjamin Roberts
Jeremy Roenick
Tomos Forrest
Rolf Mowatt Larssen
Pat Chargot
Amber Domoradzki
Brandon Neice
Aaron James
Arthur Atchabahian
Christopher Pike
Paul Chiasson
Phil Boyle
Carl Vernon
Garo Yepremian
Cosmas Inyang
Goodman Publishing
Abigail Hair
Dawson Church
Uri Bram
Dan Werb
Stephen A Mitchell
Alvin Alexander
Thomas Wilson
Jim Morekis
Tali Edut
Kenn Kaufman
Achref Hassini
Vikas Kakwani
Abhishek V R
Stephen Abbott
Andrew Campanella
Christopher Monahan
Laura Peyton Roberts
Michael N Mitchell
Gisle Solhaug
Teresa Finney
Michelle Obama
Drew Harris
Ron Hotchkiss
Leigh Bardugo
Rose Ann Hudson
Laura Sebastian
Linford Stutzman
Debbie Ford
Aaron Blight
Christopher Knight
Randy Walker
Abinash Das
Rachel Caine
Lynn Alley
Adam J Rosh
Aaron Reed Msn Crna
Mary Roach
Tomi Adeyemi
Alexander Nehamas
Deborah Madison
Blake D Bauer
Achusim Michael
Collins Kids
Domenica Marchetti
Bruce Van Brunt
Sorin Dumitrascu
Richard Langer
Kaylynn Flanders
Colin Hunter
Curt Lader
John D Gordon
Aaron Reed
Randi Hutter Epstein
Jessica Hepburn
Michael Egan
Brigitte Jordan
Abbi Glines
Richelle Mead
Laurie Chaikind Mcnulty Lcsw C
Elisabetta Viggiani
Aaron Mahnke
Scott Meyers
E P Marcellin
Sean Mcmanus
Ian Mcleod
Abu Mussab Wajdi Akkari
Linda Sarris
Chiara Giuliani
Jennifer Finney Boylan
Abdelkader Nouiri
Ada Calhoun
Catherine Ryan Hyde
T L Christianson
Andy Couturier
Andrew Skurka
Thubten Yeshe
David G Brown
Louise Thaden
Aaron Mccargo
Scott Carney
Alicia C Simpson
Dr Robert Pasahow
Lee Sandlin
Sam Jarman
Ann Frederick
Heather Lynn
Jessie Hartland
Joy Neighbors
Scott Shaw
Ben Cohen
Corinne Andrews
Stephen R Lawhead
Kayla Cottingham
Lynn Rush
Charles Fernyhough
Tom Rosenbauer
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!
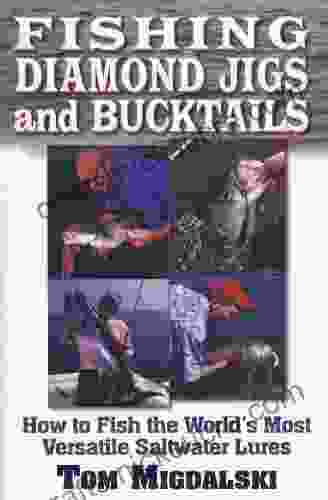

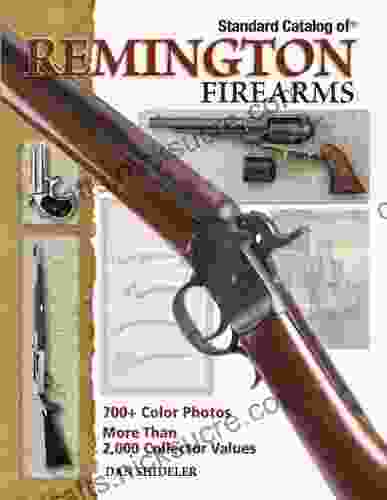

- Gus HayesFollow ·4.5k
- Billy FosterFollow ·18.3k
- Jason HayesFollow ·2k
- Dan BrownFollow ·5.7k
- Colin RichardsonFollow ·19.2k
- Adam HayesFollow ·11.3k
- Gilbert CoxFollow ·8.9k
- Chase SimmonsFollow ·16.6k
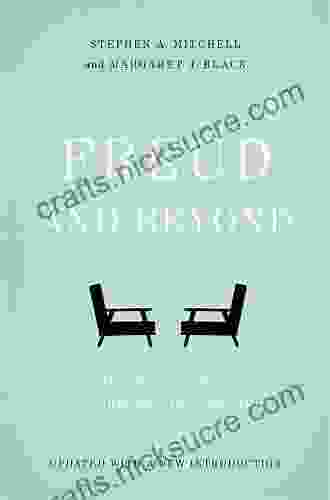

Tracing the Evolution of Modern Psychoanalytic Thought:...
Psychoanalysis, once considered a radical...
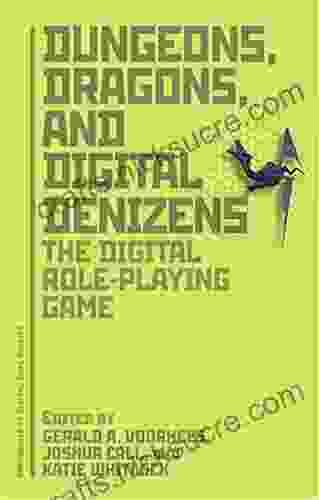

The Digital Role Playing Game Approaches To Digital Game...
These are just a few of the many...
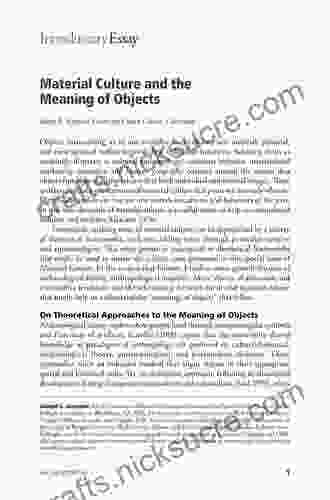

History from Things: Essays on Material Culture
History from Things:...
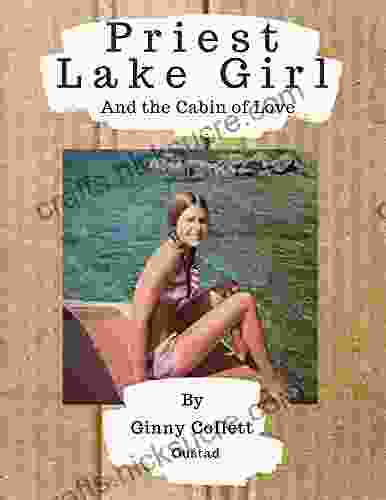

The Priest Lake Girl and the Cabin of Love: A True Story...
The Murder On...
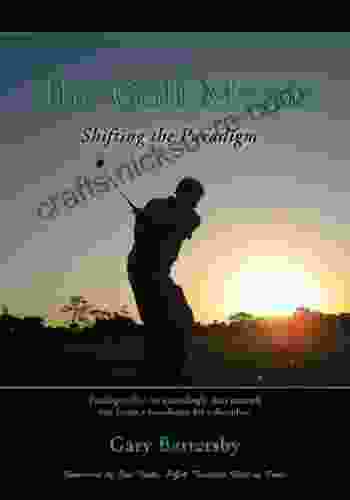

The Golf Mystic: Dick Edie's Unconventional Approach to...
In the annals of golf history, the name Dick...
4.4 out of 5
Language | : | English |
File size | : | 13336 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 307 pages |