The Complete Guide to Programming Reliable and Efficient Software Using Go

Go, also known as Golang, is a modern and efficient programming language that has quickly gained popularity among developers for its simplicity, concurrency, and scalability. It is a compiled language that emphasizes code readability, maintainability, and performance.
4.7 out of 5
Language | : | English |
File size | : | 13554 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 1771 pages |
This comprehensive guide will provide you with everything you need to know to get started with Go and build reliable, efficient software systems. We will cover the following topics:
- The basics of Go, including its syntax, data types, and control structures
- Go's concurrency model and how to write concurrent code
- Go's standard library and how to use it to build common tasks
- Best practices for writing efficient and maintainable Go code
- How to test and debug Go programs
- How to use Go to build real-world software systems
Getting Started with Go
To get started with Go, you will need to install the Go compiler and development environment. You can download the Go compiler from the official Go website.
Once you have installed Go, you can create a new Go program by creating a file with a .go
extension. For example, you can create a file named hello.go
with the following contents:
package main
import "fmt"
func main(){fmt.Println("Hello, world!") }
You can then compile this program by running the following command:
go run hello.go
This will compile and run the program, and you should see the following output:
Hello, world!
The Basics of Go
Go is a statically typed language, which means that the type of each variable must be known at compile time. Go's type system is simple and easy to understand, and it helps to prevent errors. Go also has a number of built-in types, including int
, float
, string
, and bool
.
Go's syntax is similar to that of C, but it is more modern and easier to read. Go also has a number of features that make it more productive, such as automatic memory management and garbage collection.
Go's Concurrency Model
Go is a concurrent programming language, which means that it supports multiple threads of execution. This makes it possible to write programs that can take advantage of multiple CPUs.
Go's concurrency model is based on goroutines, which are lightweight threads of execution. Goroutines are created using the go
keyword, and they can be scheduled to run on any of the available CPUs.
Goroutines are a powerful tool for writing concurrent programs, but they must be used carefully. If you are not careful, goroutines can lead to race conditions and other concurrency bugs.
Go's Standard Library
Go's standard library is a collection of packages that provide common functionality. The standard library includes packages for working with files, networks, databases, and more.
The standard library is a valuable resource for Go programmers, and it can help you to write efficient and maintainable code.
Best Practices for Writing Efficient and Maintainable Go Code
Here are some best practices for writing efficient and maintainable Go code:
- Use the correct data types for your variables.
- Avoid unnecessary allocations.
- Use goroutines to take advantage of multiple CPUs.
- Keep your functions short and simple.
- Use descriptive variable names.
- Document your code.
Testing and Debugging Go Programs
Testing is an important part of software development, and it is essential for writing reliable and efficient software. Go has a built-in testing framework that makes it easy to write and run tests.
To write a test, create a file with a _test.go
extension. For example, you can create a file named hello_test.go
with the following contents:
package main
import "testing"
func TestHello(t *testing.T){got := Hello() want :="Hello, world!"
if got != want { t.Errorf("Hello() = %q, want %q", got, want) }}
You can then run this test by running the following command:
go test hello_test.go
This will compile and run the test, and you should see the following output:
PASS ok main 0.000s
If the test fails, you will see an error message. The error message will tell you what went wrong, and it will help you to fix the problem.
Using Go to Build Real-World Software Systems
Go is a powerful language that can be used to build a wide variety of software systems. Here are some examples of real-world software systems that have been built using Go:
- Google Cloud Platform
- Docker
- Kubernetes
- InfluxDB
- Prometheus
These are just a few examples of the many software systems that have been built using Go. Go is a versatile language that can be used to build a wide range of applications, from small scripts to large-scale distributed systems.
Go is a modern and efficient programming language that is well-suited for building reliable and scalable software systems. Go is easy to learn and use, and it has a number of features that make it productive and efficient.
If you are looking for a language to use for your next software project, I encourage you to consider using Go. Go is a powerful and versatile language that can help you to build high-quality software systems.
4.7 out of 5
Language | : | English |
File size | : | 13554 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 1771 pages |
Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
Fiction
Non Fiction
Romance
Mystery
Thriller
SciFi
Fantasy
Horror
Biography
Selfhelp
Business
History
Classics
Poetry
Childrens
Young Adult
Educational
Cooking
Travel
Lifestyle
Spirituality
Health
Fitness
Technology
Science
Arts
Crafts
DIY
Gardening
Petcare
Arthur Turrell
Sheldon Axler
Laurie Chaikind Mcnulty Lcsw C
An American Citizen
Conrad Bauer
Phil Boyle
Mary Wong
Charles H Kraft
Deborah Beck Busis
Stephen Gray
Man Kam Lo
Lucio Russo
Abby Sunderland
Adam Enaz
Kemi Iwalesin
Stephen A Mitchell
Katie Lear
Baba Ifa Karade
Zoe Hamlet Silva
Emily Suzanne Clark
Tomos Forrest
Carley Roney
Abu Mussab Wajdi Akkari
Robert Allans
Randi Hutter Epstein
Linda Sarris
J Robert King
Laura Gao
Al Baird
Marc Dando
Ed Engle
Lavinia Collins
Adam Benshea
Abhishek V R
Steve Griffith
Iain Highfield
Abinash Das
Chiara Giuliani
Casey Robson
Matt Owens
Beth Newell
Scott Meyers
Sorin Dumitrascu
Rob Gray
Wayne Westcott
Lisa Heffernan
Tom Rosenbauer
Elena Aguilar
Ben Cohen
Peter Sagal
Abby Mcallister
Tomi Adeyemi
Claire Santry
Og Mandino
Al Barkow
Louise Thaden
Adam Boduch
Sasha Abramsky
Dan Morris
Programming Languages Academy
Dr Robert Pasahow
Maureen Duffin Ward
Ariana Eagleton
Xiuhtezcatl Martinez
Ian Davis
Carmen Moreno
Aaron Reed Msn Crna
Rex Ogle
Craig Timberg
E P Marcellin
Michel Roy
Michael Gruenbaum
Jimmy Houston
David Winner
Garo Yepremian
Benjamin Roberts
Scott Butler
Abigail Marshall
M J Parisian
Emily J Taylor
Christophe Jaffrelot
Louise Pickford
Abraham Silberschatz
Abbi Glines
Chris Worfolk
Abby Hafer
Abigail Melton
Aaron Graves
Richelle Mead
Maxine Levaren
Vikas Kakwani
Terry Palechuk
Christian Beamish
Neil Oliver
Vince Kotchian
Dan Werb
Randy Walker
Jeremy Paxman
Kit Bauman
Al Yellon
Richard Langer
Brad Brewer
Ron Jones
Jessica Hepburn
Michelle Obama
Adam Galinsky
Sheri Van Dijk
Thubten Yeshe
Caroline Kaufman
Tom Jackson
Kate Rope
Aaron Mahnke
Andy Couturier
Sally Clarkson
Ken Springer
Tali Edut
Kaylynn Flanders
Katrina Kahler
Michael Baigent
Lynn Alley
Daphne Poltz
Felice Fox
Irene Lewis Mccormick
Jack Cavanaugh
Aaron Mccargo
Joe Simpson
Liza Angela
Kim Gosselin
Stacy Eaton
Jim Morekis
Michael N Mitchell
Christopher Pike
Timothy Phelps
Meg Keys
Sarah K L Wilson
Phong Thong Dang
Martha Gellhorn
Stephen Abbott
Jon Butterworth
Richard Sattora
Emily K Neuburger
Alexander Nehamas
Daniel Carter Beard
Devika Primic
Barbara Decker
Israel Finkelstein
Blake D Bauer
Asato Asato
Peter Harrison
Gail Buckland
Paula Deen
Brian Hoggard
Fumio Sasaki
Suzanne Corkin
Chella Quint
Charles C Patrick
Marvin Valerie Georgia
Rory Miller
Tim Larkin
Alicia C Simpson
Judi Kesselman Turkel
Aiden Thomas
Adam Becker
Sam Jarman
Clara Shaper
Horace Kephart
Stuart Firestein
Carl Jones
Paul Mclerran
Pearson Education
Debbie Elicksen
Brian Greene
Ned Johnson
Seth Kugel
Rosie Pope
Bobby Reyes
Kenn Kaufman
Sarah Moore
Farah Shabazz Ii
P S Page
Rolf Mowatt Larssen
William Deresiewicz
Winston Chang
James Surowiecki
Jacob Neumann
Adam D Scott
Jack Moore
Adam Braus
Teresa Finney
Rachael Ray
Moon Ho Jung
Miranda Kenneally
Miriam Forman Brunell
Chris Jericho
Cassandra Eason
Axie Oh
Marie Cirano
T L Lowery
Lindsey Ellison
Aaron Oster
Randall Hyde
Leigh Bardugo
Jessie Hartland
Barbara Fox
Larry Carpenter
Ralph Villiger
Alifya And Umesh Mohite
Ada Calhoun
Annie Nicholas
Gregor Clark
Christopher Monahan
Dick Edie
Sean Lewis
Barry Dainton
Gavin D J Harper
Andrew Campanella
Elisabetta Viggiani
R L Stine
Dawson Church
Reinette Biggs
Matt Warshaw
Deborah Madison
Eze Ugbor
Sean Mcmanus
Sherri Granato
Zoyla Arana
George J Hademenos
Abigail Alling
Brandon Neice
Goodman Publishing
Jeff Bauman
Nancy Mohrbacher
Eduardo Duran
Vivienne Sanders
Mikki Daughtry
Jennifer Finney Boylan
Peter David
Abigail Hing Wen
Colin Hunter
Lois G Schwoerer
Achref Hassini
Steve Mcmenamin
Michael Crawley
Vivian Gussin Paley
Aliza Green
Aaron Lee Johnson
Lisa Robertson
Richard Lighthouse
Darrin Bergman
James Heberd
Rick Sekuloski
David Rensin
Collins Kids
David G Brown
Todd Graves
Lisa M Schab
Carl Vernon
Gil Capps
Virginia Smith Harvey
Scott Parsons
Andrea M Nelson Royes
Sophie Mccartney
Jeremy Roenick
David Warriner
Pat Chargot
Abridged Ed Edition Kindle Edition
Jason Korol
Dmv Test Bank
Ann Frederick
Phil Gaimon
David Simon
Alicia Silverstone
Lynn E Ponton
Walter Browder
Sheila Lamb
Aaron J Perry
Charles Fernyhough
Gayle Forman
Mark Januszewski
Justin Driver
Harley Rustad
Adam J Rosh
Martha C Nussbaum
Adam H Balen
Paul Chiasson
Helen Scales
Gloria Atanmo
Steven Alan Childress
Megan Lane
Barbara A Lewis
Steve Rosenberg
Ann Mariah Cook
Carolyn Jessop
Al Desetta
Sam J Miller
Rose Ann Hudson
Achille Rubini
Lin Wellford
Abigail Hair
Adam Chandler
Allan Sand
Chaz Scoggins
Michael L Bloomquist
Barbara Natterson Horowitz
Christopher S Stewart
Catherine Ryan Hyde
Bathroom Readers Institute
Amber Fox
Achim K Krull
Emma Crewe
Stefan Ball
Michelle Hodkin
Douglas W Hubbard
Natasha Preston
John Caig
George John Romanes
Jennifer Shannon
David Lipsky
Shannon Hale
Kirk Bailey
Brigitte Jordan
Scott Carney
Abdelkader Nouiri
Sarah Luddington
Genius Reads
Adam Lashinsky
Dan Shideler
Tony Soper
Amelia Whitmore
Lillian Cumic
Kel Carpenter
Jim Willis
Laurence Steinberg
Ronald Kaine
Scott Shaw
Debbie Ford
Irakli Makharadze
Chashiree M
Jay Ruud
John Kean
Amber Domoradzki
Jane Gross
Ilsa J Bick
Mary Roach
Shari Eskenas
Christina Hoff Sommers
Tom Mccarthy
Laura Peyton Roberts
Gerry Donohue
Robert Fritz
Erik Scott De Bie
Susan Zeppieri
Erika Fatland
Renda Dionne Madrigal
G Bailey
Barry Robinson
William Hamilton Gibson
Domenica Marchetti
Jennifer Greene
Michael Egan
Andrew Skurka
Ron Hotchkiss
Stephen R Lawhead
Charles Thomas Jr
Anthony Wilkenson
Charles L Byrne
Nick Holt
Bill Loguidice
Cornel West
Adam J Cox
Aaron Hahn
Stephanie Puglisi
Gisle Solhaug
Laura Sebastian
Glen Finland
Drew Harris
Chuck Callaway
Stephanie Zeiss
Bill Mckibben
Michael Johnson
Adam Freeman
Sharon Boyd
Christopher Knight
Laura Ray
Kathy Koch Phd
Roman Dial
Tamora Pierce
Aaron Blight
O Thomas Gift
Arthur Atchabahian
Gerald A Voorhees
Sarah Maslin Nir
Andy Dumas
Heather Lynn
Abby Haight
Jennifer Ackerman
Abigail Pogrebin
Paul Schneider
Rachel Caine
Kayla Cottingham
Lisa M Given
Helen C Rountree
Adam Frank
Jasna Tuta
Winifred Conkling
Monica Sorrenson
Kristin Berry
Adam Koch
Richard Martin
Brian L Gorman
Lisa Latimer
Alan Robertson
T L Christianson
Robert Reid
Betsy Miller
Abdul Foster
Bruce Van Brunt
Jeremy Shinewald
Claire Ahn
Amby Burfoot
Thomas Wilson
Abbas Kazerooni
Rania Abouzeid
Sissy Goff
Pamela Druckerman
Norton Juster
Charlie Barker
Tom Migdalski
Mark Strom
Lee Sandlin
Hillary Allen
David Feddes
Stephen Brennan
Eugene H Merrill
Achusim Michael
Joy Neighbors
Ryan Smithson
Linford Stutzman
Aaron Reed
Edward J Larson
Lisa Pineda
Fred Fields
Jenny Han
John D Gordon
Jeremy Miles
John Hancock
John Taylor
Gladys Chepkirui Ngetich
Maya Lang
Uri Bram
Shuai Huang
Kevin A Morrison
Tim Jarvis
Abbey Curran
Curt Lader
Alvin Alexander
Cosmas Inyang
Taylan Hoca
Garrett Mcnamara
Ian Mcleod
Jay Dawes
Marlene Wagman Geller
Susan Walton
Christopher Black
Ken Retallic
Aaron James
Lenyfer Garrido
Papus
Melodie M Davis
Lynn Rush
Agustin Fuentes
Abigail Tucker
Corinne Andrews
Gary Sakuma
Adam Kimelman
Adam Lazarus
Lucy Letcher
Jennifer L Hunt
Abigail Owen
Steve Roper
Hans C Ohanian
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!
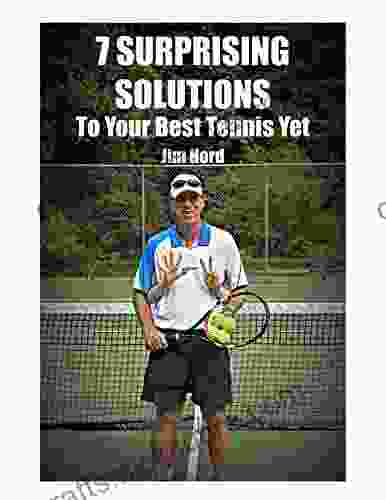

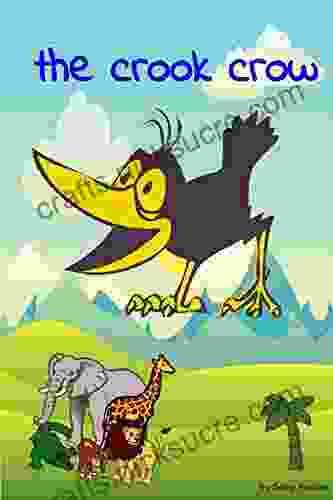

- Ivan CoxFollow ·16.4k
- Jorge AmadoFollow ·10k
- Neil ParkerFollow ·18.5k
- Geoffrey BlairFollow ·14.2k
- Gabriel HayesFollow ·16.6k
- Seth HayesFollow ·15.1k
- Eliot FosterFollow ·8.2k
- Allen GinsbergFollow ·11.5k
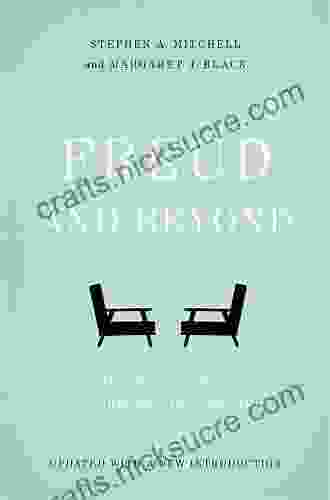

Tracing the Evolution of Modern Psychoanalytic Thought:...
Psychoanalysis, once considered a radical...
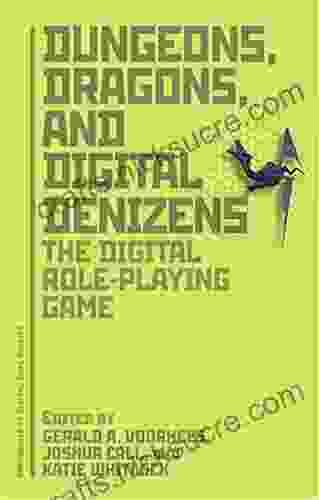

The Digital Role Playing Game Approaches To Digital Game...
These are just a few of the many...
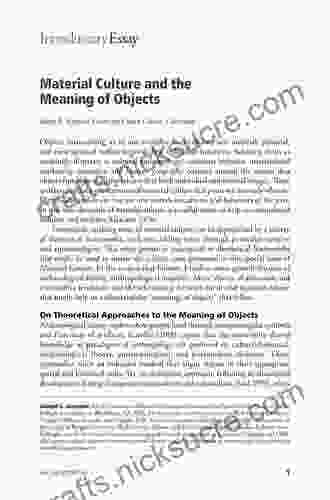

History from Things: Essays on Material Culture
History from Things:...
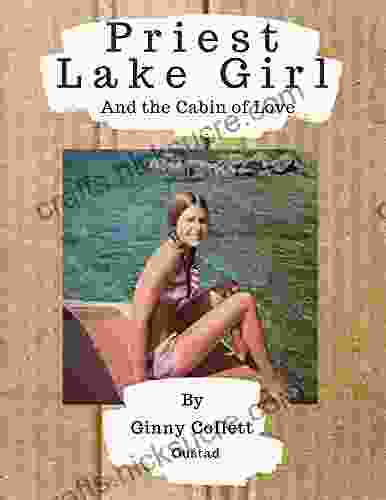

The Priest Lake Girl and the Cabin of Love: A True Story...
The Murder On...
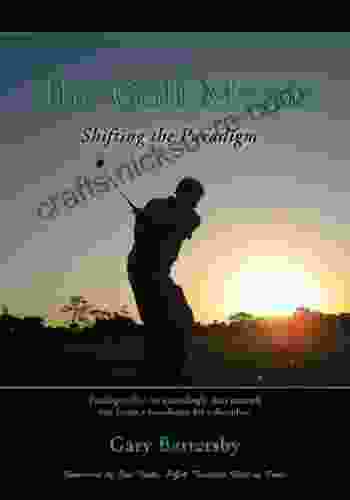

The Golf Mystic: Dick Edie's Unconventional Approach to...
In the annals of golf history, the name Dick...
4.7 out of 5
Language | : | English |
File size | : | 13554 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 1771 pages |